Tap handling, object selection, annotation¶
After having set up our basic environment with the Deep Map™ SDK, we will now look at simple map interaction methods.
To do this, we are first going to create our own MapFragment that extends the SDK MapFragment:
Java
import com.hdm_i.dm.android.mapsdk.HDMFeature;
import com.hdm_i.dm.android.mapsdk.HDMVec3;
import com.hdm_i.dm.android.mapsdk.MapFragment;
import com.hdm_i.dm.android.mapsdk.MapView;
import java.util.List;
public class MyMapFragment extends MapFragment implements MapView.TapDelegate {
@Override
public void onSingleTap(List<HDMFeature> features, HDMVec3 tapCoordinateWGS84) {
}
@Override
public void onLongPress(List<HDMFeature> features, HDMVec3 tapCoordinateWGS84) {
}
}
To use our new MyMapFragment in our demo app, we have to set it in the layout and the Activity:
Java
<fragment android:name="tutorial.hdm_i.com.myapplication.MyMapFragment"
android:id="@+id/deepmap_fragment"
android:layout_width="368dp"
android:layout_height="495dp"
tools:layout_editor_absoluteY="8dp"
tools:layout_editor_absoluteX="8dp" />
Java
//MainActivity.java
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
final MyMapFragment mapFragment = (MyMapFragment) getFragmentManager().findFragmentById(R.id.deepmap_fragment);
mapFragment.loadMap(new MapView.ReadyDelegate() {
@Override
public void onReady(MapView mapView) {
mapView.setTapDelegate(mapFragment);
}
});
}
}
As you can see, our MyMapFragment already implements the MapView.TapDelegate interface. This interface has two methods, onSingleTap and onLongPress. Both methods have the same parameters:
features: is a list of all features at the tapped location in order from top to bottom
tapCoordinateWGS84: the coordinate of the tapped point on the map in the WGS84 reference system.
Now let’s implement a simple object selection by using the onSingleTap method:
Java
//MyMapFragment.java
@Override
public void onSingleTap(List<HDMFeature> features, HDMVec3 tapCoordinateWGS84) {
if(features.size() > 0){
mapView.selectFeatureWithId(features.get(0).getFeatureId());
}
}
When you launch the app again, you can now select objects by touching them:
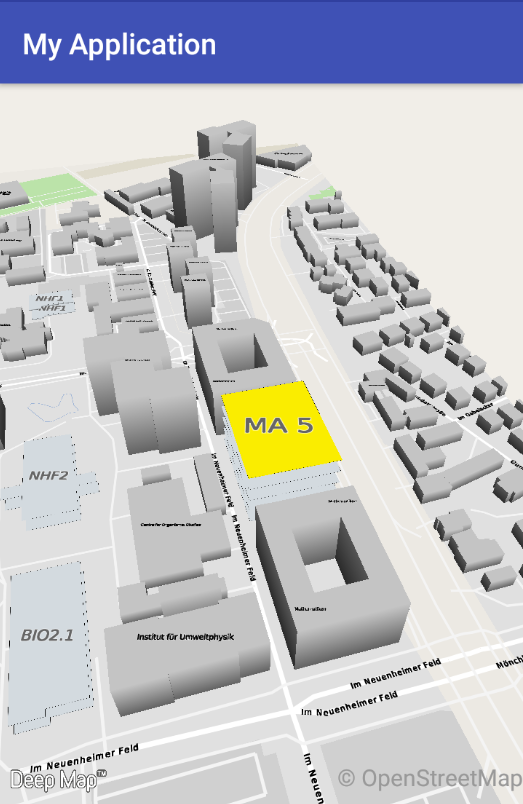
Annotations¶
For the final part of this tutorial, we will add a simple annotation to our selected feature:
This is done by adding just two lines of code to our onSingleTap method:
Java
//MyMapFragment.java
@Override
public void onSingleTap(List<HDMFeature> features, HDMVec3 tapCoordinateWGS84) {
if(features.size() > 0){
mapView.selectFeatureWithId(features.get(0).getFeatureId());
//Add a new annotation to the map:
Annotation annotation = new Annotation("Hello World!",features.get(0));
mapView.addAnnotation(annotation);
}
}
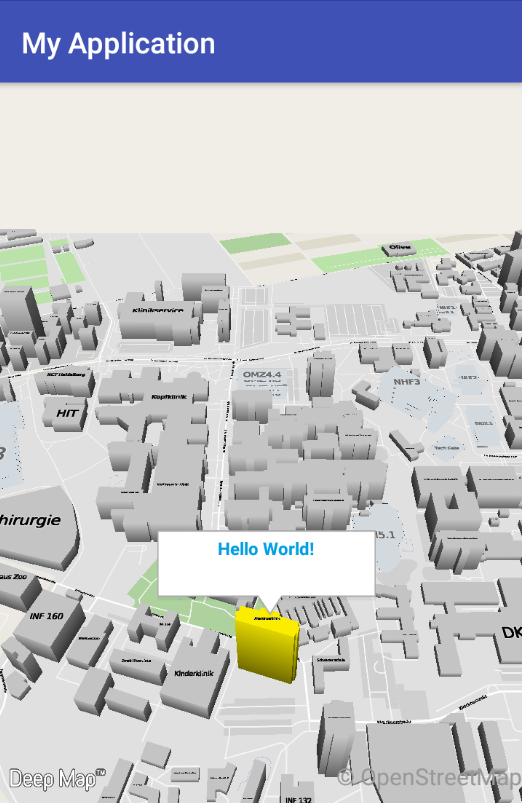
That’s it! You are now able to select objects and add annotations to the map!